Vue.js - How to handle multiple subdomains on a single app.
In this article, I’m going to show you how we can divide a Vue.js application into multiple subdomains (or multiple domains if you want) like example.com, admin.example.com, test.example.com etc.
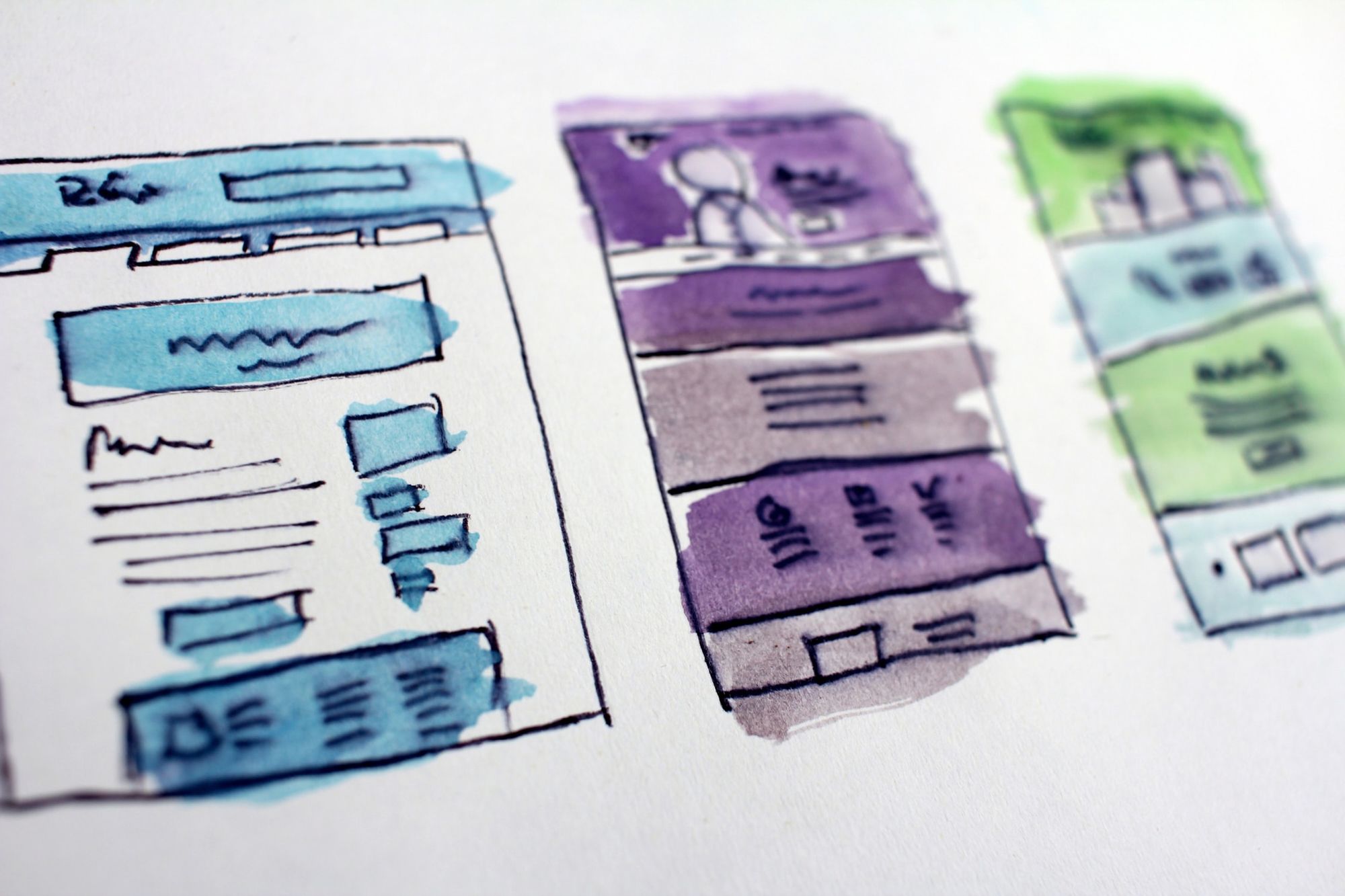
In this article, I’m going to show you how we can divide a Vue.js application into multiple subdomains (or multiple domains if you want) like example.com
, admin.example.com
, test.example.com
etc. in 3 simple steps.
- Setting up a Vue.js application with Vue Router.
- Configuring hosts on Windows and Mac/Linux for testing locally.
- Splitting the Routes and Layouts, and testing.
Github Repository:
https://github.com/apal21/Vue.js-VueRouter-multiple-subdomains
Setting up a Vue.js Application
- To set up a Vue.js application, first, we need to install the
vue-cli
globally.
$ npm install -g @vue/cli
- Now we have to initialize a new Vue application using
vue-cli
. We’ll use PWA (Progressive Web Application) boilerplate. To initialize this, run the following command:
$ vue init pwa project-name
$ cd project-name
$ npm install
$ npm run dev
- In this installation, it will ask you to install
VueRouter
. - If you didn’t install
VueRouter
from this installation or you’re using any other Vue boilerplate just run the following command to install it.
$ npm install vue-router --save
Configuring localhosts / Servers
We’re going to create 3 different domains/subdomains, myapp.localroute1.myapp.local
route2.myapp.local
on Windows and Mac/Linux.
Windows
- Open CMD (command prompt) as an administrator and run the following command:
> notepad c:\Windows\System32\Drivers\etc\hosts
- Now add these lines at the end of the file:
127.0.0.1 myapp.local
127.0.0.1 route1.myapp.local
127.0.0.1 route2.myapp.local
- Save and close the file and that’s it.
Mac/Linux
- For Mac or Linux users, open a terminal and run the following command:
$ sudo nano /etc/hosts
- Now add these lines at the end of the file.
127.0.0.1 myapp.local
127.0.0.1 route1.myapp.local
127.0.0.1 route2.myapp.local
- Press
Ctrl + X
orCommand + X
to exit the nano editor and hitY
to save the changes we’ve made in that file.
Writing the Code — Setting up VueRouter
This Github repo is the working example of this blog and you can read the README.md
file to configure the project.
Setup Routes
If you see the router
directory, it shows 3 different routes:
All three routes are handling different components.
Configure Routes
This file in the Github repo has three routes configured in it.

Here, the constant router
first fetches the URL and extracts the domain name. It checks for the domain name and assigns a route and returns it and this router
is handled by the Vue
at the bottom.
Note: You can’t use vue-router
to change the subdomain. It depends on the JavaScript’s History API
and it doesn’t let you update the cross-domain URL.
You can use HTML’s anchor tag <a>
or JavaScript’s window.location
or window.open
etc to change the subdomain.