Next.js — Make your own Progress bar indicator component easily!!!
In this article, we’ll make a Next.js component which can easily be modified for all kinds of usage using NProgress package, and use it inside our Next.js app.
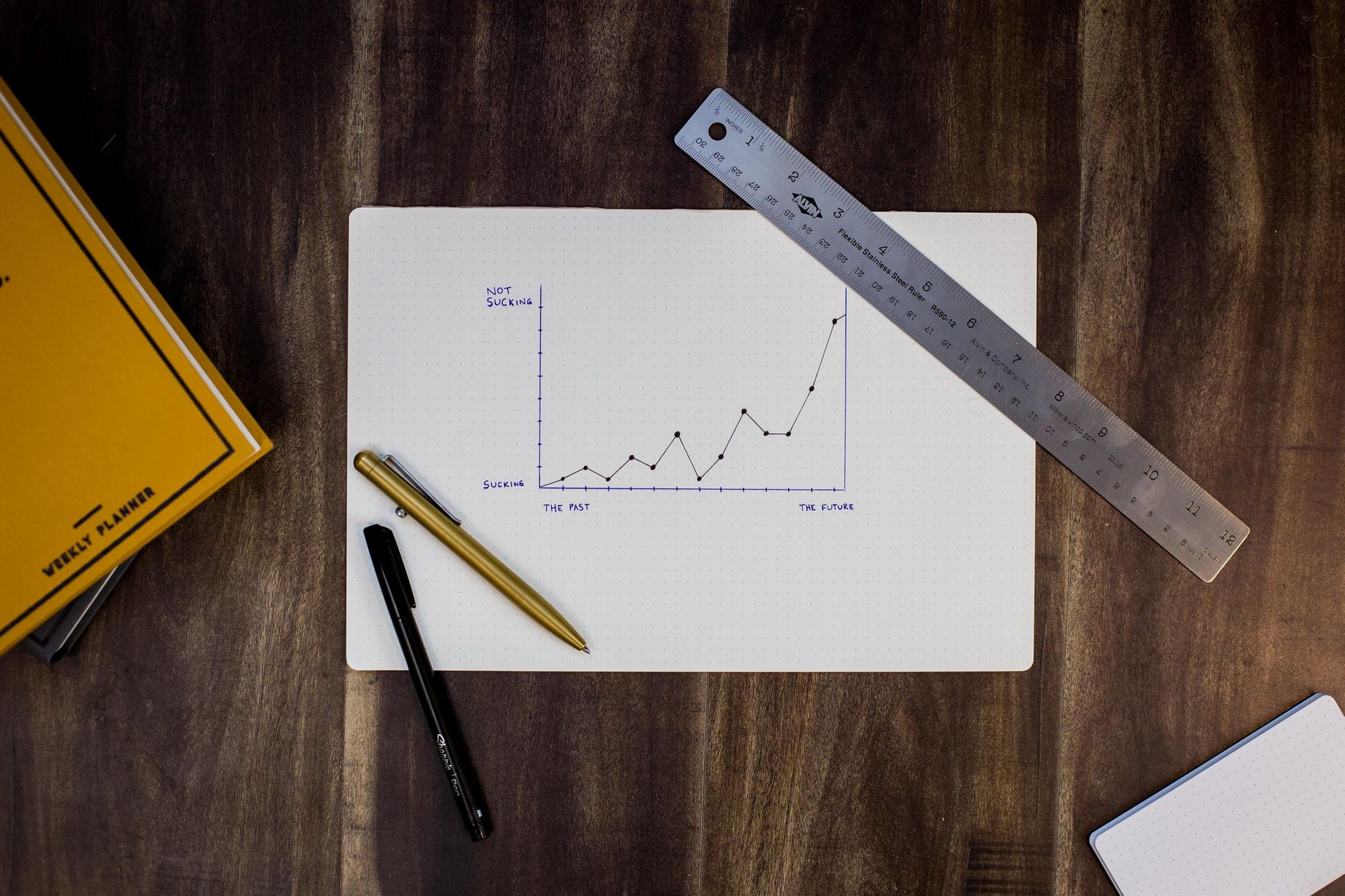
In this article, we’ll make a Next.js component which can easily be modified for all kinds of usage using NProgress package, and use it inside our Next.js app.
If you are building a Next.js application or any Server Side Rendering (SSR) app, it looks cool if there is a progress bar which shows the progress while loading a new page. I am currently(Jan 2019) building a Next.js boilerplate for my new apps and I wanted to add a progress bar component because if I don’t make a component which can be modified easily by passing the props, it would be useless because I have to re-do all the work for my all the new apps. So I ended up creating an NPM package.
If you don’t want any hassle, just import this package and see the description for the usage.
npm i nextjs-progressbar

What you should know before making this component
I expect you to know what is Next.js, React.js, Server Side Rendering (SSR) and how to make a custom <App>
in Next.js.
Installing the packages
For making this progress bar, we’ll use NProgress.
npm i nprogress

Code
Splitting the Code
Importing the packages
Create an index.js
file and import:
React
: For creating componentsNProgress
: For displaying the progress barNext Router
: For handling all the changes in routes/router.PropTypes
: For validating the props. (optional)
Creating a Component
First, we have to include the CSS of the NProgress package. We can use CDNs but we want to make this component reusable. So, I just copied the CSS and added it inside the render
method. As you already know it is too simple to add CSS in render method in Next.js.
Setting the Default Props

color
: To set the color of the progress bar. (Can take HEX, RGB or RGBA input)startPosition
: To set the starting position of the progress bar. (0.3=30%)stopDelayMs
: After changing the route(or if there is an error in the router), It removes the progress bar after this delay in ms.height
: To change the height of the progress bar. (height in px)
Configuring NProgress and Adding Router events — routeChangeStart, routeChangeComplete & routeChangeError
routesChangeStart
: This method sets the starting position of the progress bar and it starts increasing the length of the progress bar according to the options provided to it.routesChangeComplete
&routesChangeError
: For these events, we first clear all the existing timers we set previously(it matters a lot if you set a large value for the timer) and set a new timer which removes the progress bar after the specified time period.
We set all these Router Events
and other options
(which are passed using props) in componentDidMount
method (You must know the reason behind this).
Setting the PropTypes (optional)
NextNProgress.propTypes = {
color: PropTypes.string,
startPosition: PropTypes.number,
stopDelayMs: PropTypes.number,
options: PropTypes.object,
};
As you can see it is quite simple and straightforward. You add a new property to your class and specify the expected data types of the props you receive. It is not mandatory but it is a good practice if you’re planning to push your code in production.
Adding our Component in a Next.js app
For adding this component for all the pages in your Next app, you should include it inside your _app.js
file in pages
folder. I guess that the render method of your _app.js
file would look something like this:
<Container>
// other custom logic
<Component {...pageProps} />
</Container>
Now import your custom component in this file.

And… that’s it.
Change the configurations
As we discussed that we are making a component that can easily be modified and probably, you do know how to change these configurations by passing the props to this component. These are the default configurations:
<NextNProgress
color="#29D"
startPosition="0.3"
stopDelayMs="200"
height="3"
/>
If you want to add/change some more configurations, see this link, it has all the other configurations which NProgress provides itself. Example to add these configurations:
<NextNProgress
options={{ easing: 'ease', speed: 500 }}
/>
Footnotes
Let me know if you have some better ideas to make a progress bar or you want to add/remove any feature or anything. All the suggestions are appreciated.